Java 10 new features
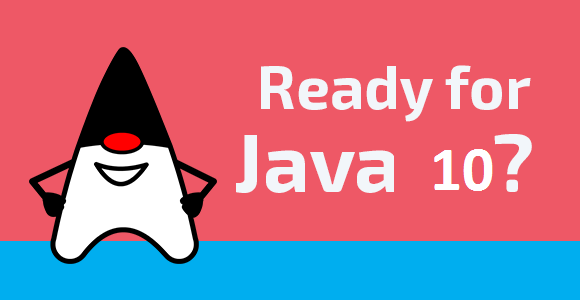
In this blog article, we will follow the new features of Java 10, released on 20 March 2018.
Java 10 syntax sugar
JEP 286: Local-Variable Type Inference
A keyword var
was introduced - type inference of local variables with initializers. A feature inspired by other languages allows skipping the type of variable during initialization.
String message = "Before Java 10";
var message = "From Java 10";
Worth mentioning that using var:
- initialization is required
- illegal use:
var message;
orvar message = null;
- var keyword can only be used to declare a local variable (e.g. inside method)
- you cannot use var to declare member variables inside the class, formal parameters, or to return the type of methods.
Unmodifiable Collections
Java classes:
- java.util.List
- java.util.Map
- java.util.Set
got a new
static method copyOf(Collection)
that returns an unmodifiable copy of the collection, for example:
List<Integer> unmodifiableList = List.copyOf(mutableList);
stream - toUnmodifiable()
Java streams got new collectors that collect unmodifiable List, Map, Set. example:
List<Integer> unmodifiableList = myList.stream()
...
.collect(Collectors.toUnmodifiableList());
Optional.orElseThrow()
By using Optional.orElseThrow
method and the value is not present, an exception NoSuchElementException
will be thrown.
It inlines to the existing Optional.orElseThrow(Supplier<? extends X> exceptionSupplier)
implementation used by consumers as an explicit alternative.
Example:
var value = myOptional.orElseThrow();
Old equivalent:
var value = myOptional.orElseThrow(NoSuchElementException::new);
Performance optimizations
JEP 307: Parallel Full GC for G1
The G1 garbage collector
is the default one since JDK 9. However, the full GC for G1
used a single-threaded mark-sweep-compact
algorithm.
This has been changed to the parallel mark-sweep-compact
algorithm in Java 10 effectively reducing the stop-the-world time during full GC.
JEP 310: Application Class-Data Sharing
In JDK 5, the feature Class-Data Sharing (CDS)
has been introduced. It allows a set of classes to be pre-processed into a shared archive file that can then be memory-mapped at runtime to reduce startup time which can also reduce dynamic memory footprint when multiple JVMs share the same archive file.
In JDK 10, the feature Application Class Data Sharing (AppCDS)
has been introduced. The idea behind AppCDS is to share
once loaded classes between JVM instances on the same host.
What is the difference?:
- Different JVM instances on the same host often load the same classes,
- CDS - store
JDK classes
into anarchive
and share it between JVM instances, - AppCDS - do the same with
application classes
andthird party library classes
.
AppCDS
can improve the footprint and runtime of your application.
Useful links: link1, link2, link3
JEP 317: Experimental Java-Based JIT Compiler
JDK 10 enables the Graal compiler, to be used as an experimental JIT compiler on the Linux/x64 platform. To enable Graal as the JIT compiler, use the following options on the java command line:
-XX:+UnlockExperimentalVMOptions -XX:+UseJVMCICompiler
Warning: it is still experimental and nobody guarantees performance improvement.
Container Awareness
JVMs are now aware of being run in a Docker container and will extract container-specific configuration instead of querying the operating system itself – it applies to data like the number of CPUs and total memory that have been allocated to the container.
You can reliably set a number of processors:
-XX:ActiveProcessorCount=count
and better control memory settings:
-XX:InitialRAMPercentage
-XX:MaxRAMPercentage
-XX:MinRAMPercentage
This feature has been backported to Java 8
as well, read here.
JEP 319: Root Certificates
With Java 10, Oracle has open-sourced the root certificates in Oracle’s Java SE Root CA program in order to make OpenJDK builds more attractive to developers and to reduce the differences between those builds and Oracle JDK builds.
Read more here
Cleanups
Tools removed:
javah
(command generates C header and source files that are needed to implement native methods) - usejavac -h
instead,policytool
(UI based tool for policy file management) - use text editor,java -Xprofoption
(option for profiling) - usejmap
.
Deprecated:
java.security.acl package
- usejava.security.Policy
instead,java.security.{Certificate,Identity,IdentityScope,Signer}
Java releases
From Java 10, it will be released every 6 months, in March and September - these are called feature releases. In the moment of release of a new feature release of Java, the older feature release is no longer supported. Long-term support release will be marked as LTS.
You can check General Availability by executing the command.
java -version
openjdk version "10" 2018-03-20
Summary
I described the most important features of Java 10. It was not a significant release, but it surprised me how many new and small improvements were added to this release.
For more detailed information I can recommend the official Java 10 release page.